What's New in Node.js v15
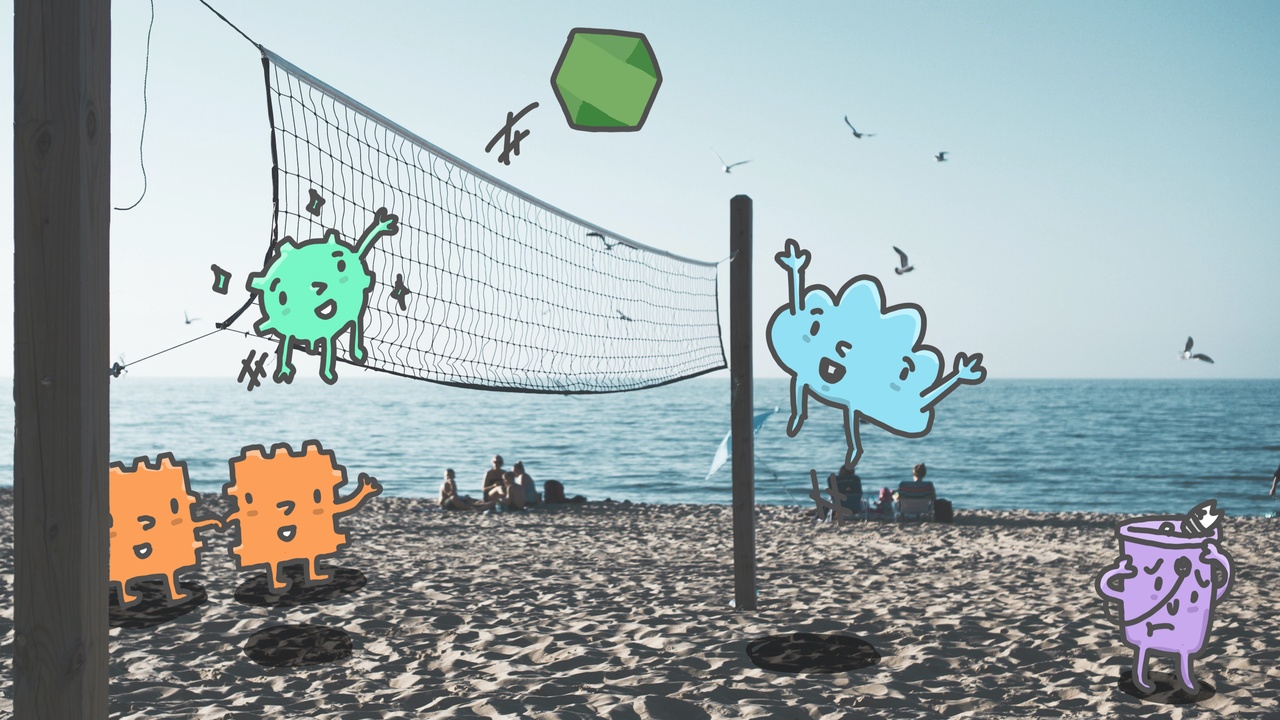
Today we are gonna talk about the newest version of the Node.js platform. Version 15 just came out in the middle of October 2020. This may surprise you, as Version 14 was released just six months earlier in April 2020. The Node.js team continues to follow their release schedule to the letter and the releases in 2020 were no different.
Version 15 is interesting because it's not an LTS version. It will reach end of life in June 2021. So don’t go deploying any of your production apps, as Version 15 is more about installing and understanding experimental features. These experimental features will then be in Version 16.
In this article, we’ll go through the newest features in Node.js v15. At the end, I’ll show you how to install this new version so you can check it out and try a few things for yourself.
If you’d prefer, you can also watch the video on YouTube:
npm Version 7
The first cool feature of Node.js v15 is npm Version 7. This new
version of the npm CLI utility is packaged with Node.js v15, but it can also be installed
separately. A new package-lock.json
format and compatibility with
yarn.lock
files are some of the new features included with npm v7. Another, and one
of the coolest imo, is npm workspaces. There’s more to npm v7 and you can read about it in the
release notes for this version.
Breaking Changes in Promises
In one of the biggest breaking changes in years, Promises that
don’t handle rejections are going to throw now, instead of just warning. If you’ve been working
with Promises or async/await in your Node.js applications, occasionally you might see unhandled
rejection errors in the console. This happens when you have a Promise or an async function, and
either don’t have a catch on the Promise (like
Promise.then().catch()
) or if the async function call isn’t wrapped in a try block. If this
occurs in Node.js v15, your program will crash completely and stop running.
This is a huge change. Previously, when Node.js programs didn’t handle Promise errors correctly there would only be a warning output. This change is going to require a lot of applications to update, especially if I’ve written any of the code. Node.js v15 gives you a chance to fix your applications before this becomes canon in Node.js v16 (released in April 2021).
Here’s how to fix this. Previously, if you did this:
myPromiseFunc.then(() => {})
You should make sure to add a
.catch()
like this:
myPromiseFunc.then(() => {}).catch(err => console.error(err))
If you are calling an async function and previously did this:
const result = await myAsyncFunc()
You should make it v15+ safe by wrapping it in a try/catch like this:
try { const result = await myAsyncFunc() } catch (err) { console.error(err) }
By updating your code with both of these formats, you can make sure that your application will be ready for the future of Node.js.
QUIC Support
One of the new experimental features is called QUIC support. You must use a flag to enable it in Node.js v15, and it is
essentially a new type of network connection. Here’s how you enable it:
node index.js --enable-quic --quic-version=h3-23
Here’s how this new feature works. HTTP and HTTPS are built on top of TCP. Your Node.js server uses a TCP connection under the hood to connect, for example to a browser. QUIC is a new type of protocol that runs on top of UDP instead of TCP. It does a lot of the things that TCP and HTTPS do together, but natively. This is supposed to be able to significantly reduce the latency involved when making network connections with HTTPS.
QUIC is experimental, and to use it widely on the internet, there needs to be a lot more support added. If the rollout of things like HTTP/2 and IPv6 give any indication, don’t expect to use it in production anytime soon.
JavaScript Language Features
Node.js v15 has also added several awesome JavaScript language features. I can see these language features being quite useful in making more succinct code that is easier to read. In Node.js v15, there’s a new version of the V8 JavaScript engine, which is used to interpret your JavaScript code. The V8 engine is what powers JavaScript in the Google Chrome Browser. So, any new JavaScript feature that’s in Chrome will be supported in Node.js v15. Here are some of the highlights of these new language features.
Promise.any()
One of the first new language features is
Promise.any()
. Here’s how to use it:
const promises = [promise1, promise2, promise3]
Promise.any(promises).then(result => console.log(result))
The
.then()
function will be executed with whichever Promise resolves first. It will only be executed once,
so the result of the other Promises will essentially be ignored.
It’s a feature that was in Bluebird for a long time, but it’s a brand new native Promise feature now in Node.js v15. You’ll be able to use it in updated Chrome browsers as well.
Also, make sure to handle a possible Promise rejection with a
.catch()
so your application doesn’t crash!
String.prototype.replaceAll()
I’m all for more native string manipulation functions in
JavaScript, so I’m glad to see the addition of
String.replaceAll()
. You probably already know about
String.replace()
, which lets you replace words, letters, and so on in a
string.
String.replaceAll()
just replaces all instances of that pattern. You can pass in a string
and then pass in a RegEx. Previously, if you wanted to replace all instances of something in a
string, you could use replace with a RegEx and then /ig
. Now, you can just use the
replaceAll()
function, and it does all those things for you.
Here’s the MDN documentation for String.prototype.replaceAll().
Logical Assignment Operators
The last new language feature is logical assignment operators. I couldn’t have even imagined these would be needed, but now I can’t help thinking of all the ways I’ll use them.
These new logical assignment operators look like this:
&&=
||=
??=
These new operators will perform an evaluation step before they assign a value to a variable.
Let’s say you have a statement like
x &&= 10
. The previous value of that
x
will
be evaluated for truthiness because of the &&=
operator. So, with
&&=
, if the previous value of
x
is
truthy, then it will have the value of
10
assigned to it. If we’ve got have the following statements:
x = 20
x &&= 10
Then
x
would
equal 10
after the second line.
In this case:
x = 0
x &&= 10
Because
0
is a
falsy value, the &&=
operator would evaluate to false and so
x
would
continue to equal 0
after the second line.
The next logical assignment operator is
||=
,
and it works with the opposite function from
&&=
. The value will be assigned to if the operator evaluates to
falsy. Here’s an example:
x = 0
x ||= 10
// x will equal 10
x ||= 20
// x will still equal 10
The final logical assignment operator is
??=
,
which will assign to the variable if the value of the variable is nullish. Nullish is a newer
term, which means it’s either
null
or
undefined
. Here’s an example:
let x
x ??= 10
// x will equal 10
x ??= 20
// x will still equal 10
Here are the MDN docs for each operator:
Logical nullish Assignment (??=
)
How to Install Node.js v15
If you’re using a Node.js version manager, such as nvm (like I do), then you can install today using the command:
nvm install 15
. You can also download an installer from the Node.js homepage.
Using a version manager for your Node.js installs is the easiest way to get the newest versions of Node.js and switch between versions when you need to. Some great options are nvm, or n. If you’re on Windows, you can try using nvm-windows. Using a version manager will make it easier for you to try out Node.js v15 without committing to it.
Thank You!
There are tons of new features in Node.js v15. All of them have become official with the release of Version 16 last month, April 2021. Try them all out to see which will have the biggest impact on your applications.